图书介绍
数据结构的C++伪码实现 英文版PDF|Epub|txt|kindle电子书版本网盘下载
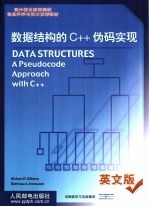
- Richard F.Gilberg,Behrouz A.Forouzan编著 著
- 出版社: 北京:人民邮电出版社
- ISBN:7115097666
- 出版时间:2002
- 标注页数:663页
- 文件大小:142MB
- 文件页数:684页
- 主题词:
PDF下载
下载说明
数据结构的C++伪码实现 英文版PDF格式电子书版下载
下载的文件为RAR压缩包。需要使用解压软件进行解压得到PDF格式图书。建议使用BT下载工具Free Download Manager进行下载,简称FDM(免费,没有广告,支持多平台)。本站资源全部打包为BT种子。所以需要使用专业的BT下载软件进行下载。如BitComet qBittorrent uTorrent等BT下载工具。迅雷目前由于本站不是热门资源。不推荐使用!后期资源热门了。安装了迅雷也可以迅雷进行下载!
(文件页数 要大于 标注页数,上中下等多册电子书除外)
注意:本站所有压缩包均有解压码: 点击下载压缩包解压工具
图书目录
1 Introduction1
1-1 Pseudocode2
Algorithm Header2
Purpose,Conditions,and Return3
Statement Numbers4
Variables4
Algorithm Analysis5
Statement Constructs5
Pseudocode Example6
1-2 The Abstract Data Type7
Data Structure8
Atomic and Composite Data8
Abstract Data Type9
1-3 A Model for an Abstract Data Type10
ADT Operations11
ADT Data Structure11
ADT Class Templates13
1-4 Algorithm Efficiency13
Linear Loops14
Logarithmic Loops14
Nested Loops15
Big-O Notation17
Standard Measures of Efficiency19
Big-O Analysis Examples20
1-5 Summary22
1-6 Practice Sets23
Exercises23
Problems25
Projects25
2 Searching27
2-1 List Searches28
Sequential Search28
Variations on Sequential Searches30
Binary Search33
Binary Search Algorithm36
Analyzing Search Algorithms37
2-2 C++ Search Algorithms38
Sequential Search in C++38
Binary Search in C++40
Search Example41
2-3 Hashed List Searches44
Basic Concepts44
Hashing Methods46
Hashing Algorithm50
2-4 Collision Resolution51
Open Addressing53
Bucket Hashing57
Linked List Resolution57
Hash List Example58
Combination Approaches58
2-5 Summary62
2-6 Practice Sets64
Exercises64
Problems65
Projects65
3 Linked Lists67
3-1 Linear List Concepts68
Insertion68
Deletion69
3-2 Linked List Concepts70
Retrieval70
Traversal70
Nodes71
Linked List Data Structure71
Pointers to Linked Lists73
3-3 Linked List Algorithms73
Create List73
Insert Node74
Delete Node78
Search List80
Retrleve Node83
Unordered List Search83
Full List84
Empty List84
List Count85
Traverse List85
Destroy List87
3-4 Processing a Linked List88
Add Node90
Remove Node90
Print List91
Testing Insert and Delete Logic92
Append Lists93
3-5 List Applications93
Array of Lists95
3-6 Complex Linked List Structures97
Circularly Linked Lists97
Doubly Linked Lists98
Multilinked Lists103
Multilinked List Insert104
Multilinked List Delete105
3-7 Building a Linked List-C++Implementation105
Data Structure105
Application Functions106
3-8 List Abstract Data Type-Linked List Implementation112
List ADT Declaration113
3-9 Summary124
3-10 Practice Sets125
Exercises125
Problems127
Projects128
4 Stacks135
4-1 Basic Stack Operations136
Push136
Pop136
Data Structure137
4-2 Stack Linked List Implementation137
Stack Top137
Stack Algorithms139
4-3 Stack Applications146
Reversing Data146
Reverse a List146
Convert Decimal to Binary147
Parsing148
Postponement149
Backtracking157
4-4 Eight Queens Problem-C++Implementation163
Get Board Size164
Main Line Logic164
4-5 Stack Abstract Data Type Implementation169
Data Structure169
Stack ADT Implementation170
4-6 Stack ADT-Array Implementation175
Array Data Structure176
Create Stack Array177
Push Stack Array178
Pop Stack Array178
Stack Top Array179
Stack Count Array180
Full Stack Array180
Empty Stack Array180
Destory Stack Array181
4-7 Summary181
4-8 Practice Sets182
Exercises182
Problems183
Projects185
5 Queues189
5-1 Queue Operations190
Enqueue190
Dequeue190
Queue Rear191
Queue Front191
Queue Example192
5-2 Queue Linked List Design192
Data Structure192
Queue Algorithms194
Create Queue194
Enqueue196
Dequeue197
Retrieving Queue Data198
Full Queue199
Empty Queue199
Queue Count200
Destroy Queue200
5-3 Queuing Theory200
5-4 Queue Applications202
Queue Simulation202
Categorizing Data209
5-5 Categorizing Data-C++Implementation211
Main Line Logic211
Fill Queues212
Print Queues213
Print One Queue214
Queue Structure215
5-6 Queue ADT-Linked List Implementation215
Queue ADT Implementation216
5-7 Queue ADT-Array Implementation221
Array Queues Implementation222
5-8 Summary228
5-9 Practice Sets229
Exercises229
Problems231
Projects232
6 Recursion237
6-1 Factorial-A Case Study238
Recursion Defined238
Recursive Solution239
Iterative Solution239
6-2 How Recursion Works240
6-3 Designing Recursive Algorithms242
The Design Methodology243
Limitations of Recursion243
Design Implementation-Reverse a Linked List244
6-4 Another Case Study-Fibonacci Numbers246
6-5 The Towers of Hanoi249
Recursive Towers Of Hanoi250
6-6 C++Implementations of Recursion253
Fibonacci Numbers253
Prefix to Postfix Conversion254
Towers of Hanoi259
6-7 Summary260
6-8 Practice Sets261
Exercises261
Problems263
Projects264
7 Introduction to Trees265
7-1 Basic Tree Concepts266
Terminology266
Tree Representation268
7-2 Binary Trees270
Properties271
Depth-First Traversals273
7-3 Binary Tree Traversals273
Breadth-First Traversals278
7-4 Expression Trees280
Infix Traversal280
Postfix Traversal281
Prefix Traversal282
7-5 General Trees282
Changing General Tree to Binary Tree282
Insertions into General Trees283
General Tree Deletions285
7-6 Huffman Code285
7-7 Summary288
7-8 Practice Sets290
Exercises290
Problems293
Projects293
8 Search Trees294
8-1 Binary Search Trees295
Definition295
Operations on Binary Search Trees296
Binary Search Tree Search Algorithms297
8-2 AVL Trees306
Balancing Trees309
AVL Balance Factor309
AVL Node Structure314
AVL Delete Algorithm319
Adjusting the Balance Factors323
8-3 AVL Tree Implementation324
Data Structure324
Program Design325
Count Words Summary328
8-4 AVL Abstract Data Type329
AVL Tree Data Structures330
AVL Tree Functions331
AVL Tree Data Processing342
AVL Tree Utility Functions344
8-5 Summary347
8-6 Practice Sets348
Exercises348
Problems350
Projects351
9 Heaps354
9-1 Heap Definition355
9-2 Heap Structure355
9-3 Basic Heap Algorithms356
ReheapUp356
ReheapDown358
9-4 Heap Data Structure360
9-5 Heap Algorithms361
ReheapUp361
ReheapDown362
BuildHeap363
InsertHeap364
DeleteHeap365
9-6 Heap Applications367
Selection Algorithms367
Priority Queues368
9-7 A Heap Program370
Heap Program Design370
Heap Functions375
9-8 Summary377
9-9 Practice Sets378
Exercises378
Problems380
Projects380
10 Multiway Trees383
10-1 m-Way Search Trees384
10-2 B-Trees385
B-Tree Insertion387
B-Tree Insert Design388
B-Tree Insert Node389
B-Tree Deletion396
Traverse B-Tree407
B-Tree Search410
10-3 Simplified B-Trees411
2-3 Tree411
2-3-4 Tree411
10-4 B-Tree Variations412
B*Tree412
B+Tree413
10-5 Lexical Search Tree413
Tries414
Utility Functions415
Header File415
Trie Structure415
10-6 B-Tree Abstract Data Type415
Insert Algorithms428
Delete Algorithms428
10-7 Summary434
10-8 Practice Sets435
Exercises435
Problems436
Projects436
11 Advanced Sorting Concepts438
Sort Order439
11-1 General Sort Concepts439
Sort Stability440
Sort Efficiency440
Passes440
11-2 Insertion Sorts441
Straight Insertion Sort441
Shell Sort443
Insertion Sort Algorithms447
Insertion Sort Implementation449
11-3 Selection Sorts451
Straight Selection Sort451
Selection Sort Algorithms456
Selection Sort Implementation457
11-4 Exchange Sorts459
Bubble Sort459
Bubble Sort Algorithm461
Quick Sort462
Exchange Sort Algorithms468
11-5 Summary470
Exchange Sort Implementation470
11-6 External Sorts474
Merging Ordered Files474
Merging Unordered Files475
The Sorting Process476
Sort Phase Revisited482
11-7 Summary484
11-8 Practice Sets485
Exercises485
Problems486
Projects486
12 Graphs490
12-1 Terminology491
12-2 Operations492
Add Vertex492
Find Vertex493
Delete Edge493
Add Edge493
Delete Vertex493
Traverse Graph494
12-3 Graph Storage Structures497
Adjacency Matrix497
Adjacency List498
12-4 Graph Algorithms499
Create Graph500
Insert Vertex500
Delete Vertex502
Insert Arc503
Delete Arc505
Retrieve Vertex506
First Arc507
Depth-First Traversal508
Breadth-First Traversal510
12-5 Networks512
Minimum Spanning Tree512
Shortest Path Algorithm517
12-6 Abstract Data Type521
Insert Vertex523
Delete Vertex524
Insert Arc525
Delete Arc526
Depth-First Traversal528
Breadth-First Traversal529
12-7 Summary531
12-8 Practice Sets532
Exercises532
Problems533
Projects534
Appendixes537
A ASCII Tables537
B Structure Charts542
C Program Standards and Styles549
D Random Numbers554
E Standard C++Libraries559
F C++Function Prototypes561
G Classes Related to Input and Output569
H The String Class574
I Pointers to Functions584
J Inheritance587
K C++Templates601
L Standard Template Library608
Solutions to Selected Exercises626
Glossary647
Index657